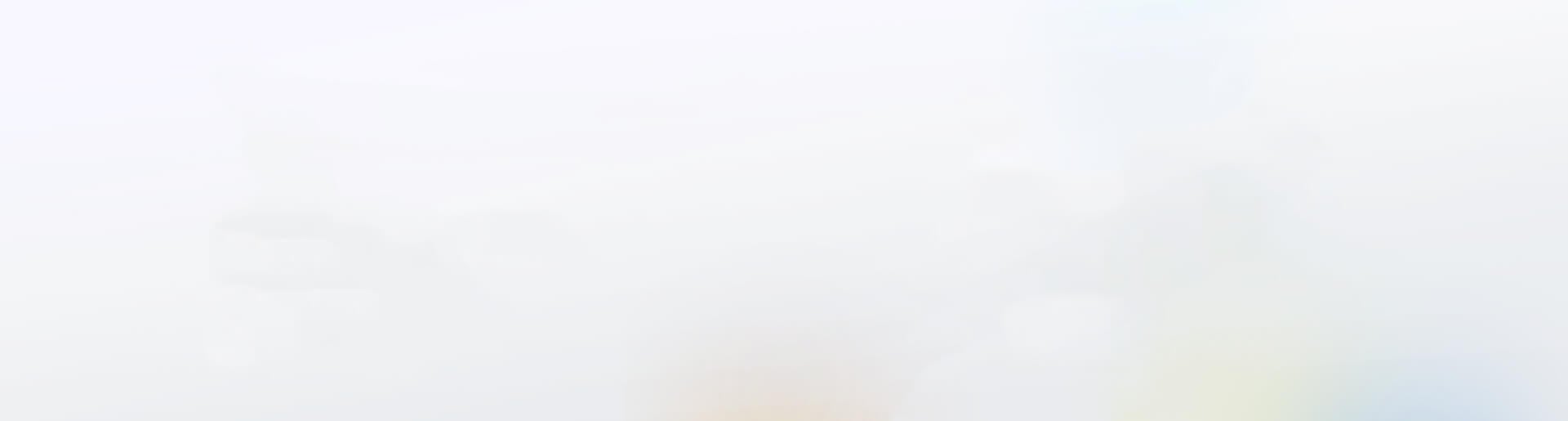
API methods determine the action performed on a resource or set of data. Learn more, plus how OpenLegacy can help with methods for legacy-based APIs
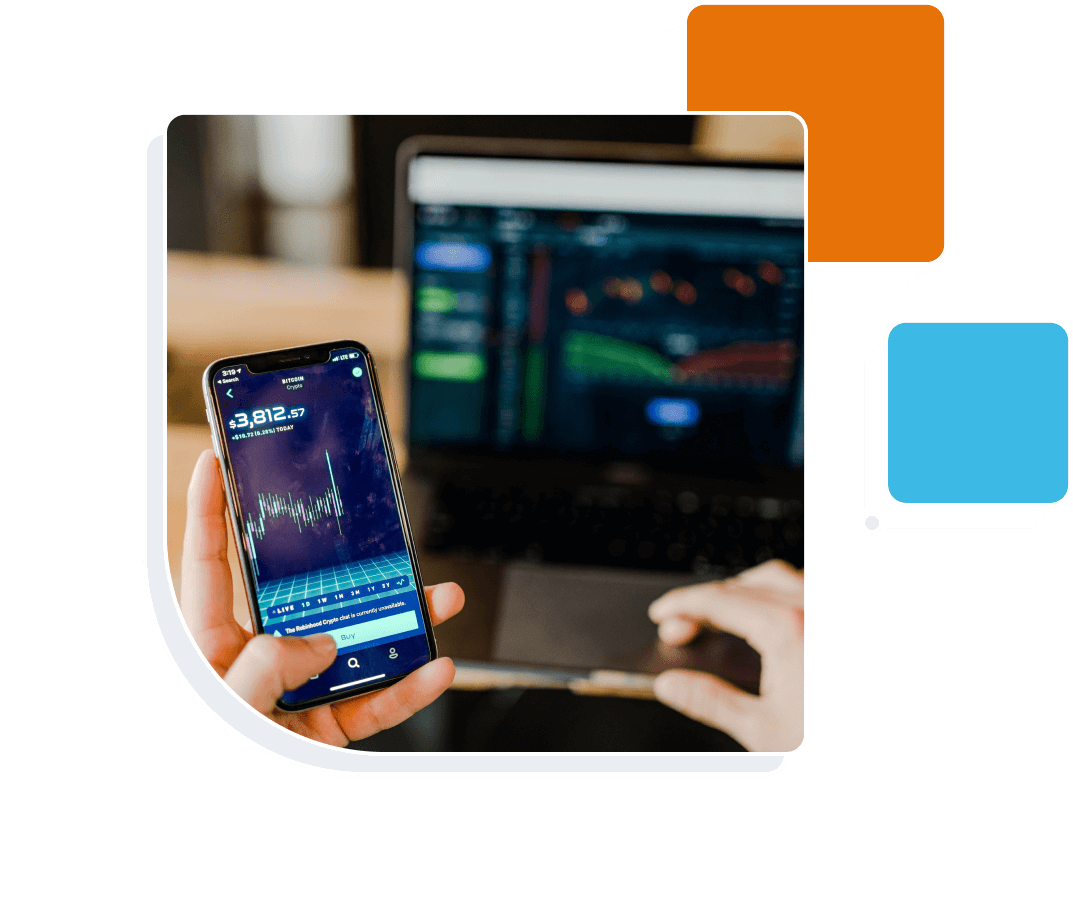
API Methods: Definition, Types, and How To Get Usable and Functional API Methods for Your Legacy Systems
An API method is a basic building block used in coding requests on API servers. It’s a fundamental element of the data exchange process, and anyone who wants to understand more about how APIs work in practice should be familiar with the concept.
Here, we’ll answer the question “what is an API method” and look at a few of the most useful ones related to RESTful APIs. We’ll also explore the challenges regarding legacy systems and API methods and explain how best to tackle them.
What are API methods?
Application programming interfaces, also known as APIs, are a reliable way for different software applications to communicate with one another. Through the use of dedicated API connectors, you can get pretty much any two or more applications to exchange data. Even if they store or process data in very different ways.
This is a broad-brush description of what APIs do, but it’s also worth looking into how they do it. And this is where we come to what’s known as an API method.
What are API methods? Simply put, they represent the different types of requests that can be made of an API endpoint to elicit the desired response.
Essentially, an API has a base URL and a number of endpoints, much like a website might have a domain and a variety of subdomains.
For instance, an API for an eCommerce platform that specializes in car parts might have an identifier:
https://cobaltrangercars.api.com/accounts
In this case, https://cobaltrangercars.api.com is the base URL of the API and, /accounts is the endpoint.
Endpoints act like gateways to different types of data resources. In the example above, /accounts will be where information about account details is stored, but other typical endpoints could include /orders and /users.
When you want to do something with the data stored in these resources, you’ll use a range of different API methods to do it, depending on what you want to achieve.
Different types of API methods
At this point, it’s important to note that the word "method" is one of those terms—like “applications” or “functions”--that are not only widely used but can also have slightly different meanings in different contexts.
Generally speaking, a method can be described as any kind of functional endpoint a consumer can interact with.
When you’re working with processes like this, it’s vital to define methods based on how the consumer understands them, rather than on the way the existing backend was designed. We’ll go into the details of why that is later on, but keep it in mind for the moment.
Often, when someone asks about API method types, they have one specific thing in mind: HTTP methods. These are relevant to one particular kind of API known as a RESTful API.
REST stands for Representational State Transfer and it describes a specific architectural approach to designing services for the web. It was introduced back in 2000 and has since become a highly popular protocol for API models because it’s so flexible.
It has the advantage of being format-agonistic, which means you can use it with all sorts of protocols, including XML, JSON, HTML, and so on. The result is that RESTful APIs tend to be both fast and lightweight. This makes them ideal for modern uses like mobile app projects and IoT devices.
Although RESTful API implementations don’t necessarily have to use HTTP as their application protocol, most of them do. That includes the methods set out below.
So, in practical terms, how do API methods work? Here’s an explainer on how to use API methods (or, specifically, HTTP methods) to achieve common data-related objectives.
GET method
The GET method is one of the simplest to understand and execute. As the name implies, you use it when you want to “get” information from a specified resource.
Let’s say you need to access information about some Cobalt Ranger Cars’ customer accounts. In this case, you’d send a GET request to the /accounts endpoint like this:
const baseURL = "https://cobaltrangercars.api.com";
fetch(`${baseURL}/accounts`)
.then(res => res.json())
.then(data => console.log(data))
.catch(error => console.log(error.message));
This returns a list of user accounts, and you’ll notice there’s a line at the end to catch any errors. This is in case there’s a problem with the request. For example, if you call the resource and the data isn’t available, that’s when you get the all-too-familiar 404 error message.
PUT method
The PUT method can either create a resource or update one already held on the API server.
There are several methods for updating data, so it’s crucial to distinguish between them depending on the intended objective. In the case of PUT, it replaces all the data that is already stored in a specified resource.
The client first of all specifies the identifier for the resource, then the body contains all the information to go into it. If the resource already exists, the data in it will be replaced with all the new information. If it doesn’t, a new resource will be created.
Here’s how an employee record could be updated to the Cobalt Ranger Cars API /employees endpoint using the PUT method:
const updatedData = {
fname: "Peter",
paymentMethod: "ACHtransfer",
rate: "40",
hours: 150,
overtimeHours: 0,
salaryAmount: "6000",
lname: "Baker",
compType: "Hourly",
}
const baseURL = "https://cobaltrangercars.api.com";
fetch(`${baseURL}/employees/5`, {
method: "PUT",
body: JSON.stringify(updatedData)
})
.then(res => res.json())
.then(data => console.log(data))
.catch(error => console.log(error));
An additional note about PUT requests, because it’s always important to bear sensible API governance principles in mind, is that it’s good practice to implement bulk PUT operations that can batch updates to multiple resources in a collection. It’s an efficient way to do it that can improve overall performance.
DELETE method
If you want to delete data, you can do it easily with the DELETE method. All it takes is setting the method to DELETE and specifying the identifier of the resource at the relevant endpoint. For instance:
const baseURL = "https://cobaltrangercars.api.com";
fetch(`${baseURL}/users/12`, {
method: "DELETE"
})
.then(res => res.json())
.then(data => console.log(data))
.catch(error => console.log(error));
If the process is successful, the web server will respond with HTTP status code 204 (No Content).
POST method
The POST method is used to send data to the API. It creates a new resource by assigning an identifier for it and then returns that identifier to the client.
It’s commonly applied to collections to add a new resource to that collection in particular. For instance, a new employee record could be added to the API using the POST method:
const newUser = {
fname: "Jennifer",
paymentMethod: "ACHtransfer",
rate: "1000",
hours: 40,
overtimeHours: 10,
salaryAmount: "4400",
lname: "Thompson",
compType: "Salaried",
}
const baseURL = "https://cobaltrangercars.api.com";
fetch(`${baseURL}/employees`, {
method: "POST",
body: JSON.stringify(newUser)
})
.then(res => res.json())
.then(data => console.log(data))
.catch(error => console.log(error));
POST doesn’t necessarily create a new resource, though. It’s also the right choice if you want to submit data for processing to an existing resource.
PATCH method
When updating records, you don’t always want to completely replace existing data or add a completely new entry. Sometimes, all you’re looking to do is update some of the data.
In this scenario, you’d use the PATCH method. You specify an object that contains items that you want to change, and the API server updates the resource data corresponding to the items you choose. For example:
const patchObj = { fname: "Carmen Oduku" };
const baseURL = "https://cobaltrangercars.api.com";
fetch(`${baseURL}/users/1`, {
method: "PATCH",
body: JSON.stringify(patchObj)
})
.then(res => res.json())
.then(data => console.log(data))
.catch(error => console.log(error));
These are five of the most commonly used API methods, and they’re generally pretty straightforward to use. However, when you’re dealing with legacy systems, there can be several complications that you need to take into account.
API methods and legacy systems: Challenges you need to overcome
The key question is: How does an API method work with legacy systems? The reality is that you can make it a success, but in the initial stages, you have to be careful about how you approach the process.
With modern architectures, API methods are usually attached to specific domains. In other words, all methods relevant to customers should be in customer domains, all methods relevant to accounts should be in accounts domains, and so on. This makes processes like API orchestration relatively straightforward, even if the underlying applications are quite complex.
However, this tidy structure isn’t what you generally see when it comes to legacy systems. That’s because in legacy systems, all the data is bundled together, which makes this kind of organized separation difficult to achieve.
Remember how we said earlier that it’s important to define methods based on how the consumer understands them and not according to the design of the backend? This is why.
All the data being bundled together like this can mean you have API methods that are mismatched, with no domains, dictated by the underlying structure of the data. What can happen is that the methods just end up looking like the underlying legacy systems and, as a result, are way too complicated to operate efficiently.
Here's an illustration of the problem in practical terms. Let’s imagine Cobalt Ranger Cars’ legacy system is parts-focused since producing auto parts is their core business.
The data that exists there will be arranged according to the characteristics of those parts by serial numbers, SKUs, etc.
Now, they want to move to a modern, customer-centric application, but that needs data from the legacy system. And, unfortunately, the old system and the new system are not well-aligned.
For instance, it will be tricky to query all the parts that an individual customer needs rather than all the customers that need an individual part.
Luckily, there are ways to deal with this. What you need is a way of abstracting things from the structure of the data.
How OpenLegacy can help
With OpenLegacy, you can detach legacy-based APIs from the way the data is structured behind the scenes. You can actually retrofit domains into your legacy application to achieve API methods that are appropriate to your needs.
So, in the example of Cobalt Ranger Cars, this could mean retrofitting customer domains to the legacy system, so that there’s a method available to request the data on all the parts a particular customer needs.
In a way, it’s a little like creating scaffolding around a building. That can give you access to the exact room you want directly from the outside without having to climb all the stairs from the ground up first.
But this isn’t the only way you can benefit from the APIzation of your legacy system. There are many other advantages as well.
Appropriate API methods are just one example of the benefits of APIzation with OpenLegacy
OpenLegacy allows you to wrap your existing legacy assets with custom APIs to unlock the value of the data while future-proofing your systems.
There’s no need to make complex code changes, either, which means you can deploy modernization tech rapidly and ensure a seamless transition without having to worry about extensive and costly downtime.
What’s more, when you convert your legacy system into microservices-based APIs, it puts you in a great position to be able to develop innovative applications quickly.
You’ll be able to mount a nimble response to sudden changes in customer expectations, market conditions, or the technological environment, so your business will always stay ahead of the pack.
In short, there’s no better way of ensuring you’ll always be able to leverage the full value of your legacy data, no matter what the future brings. And because your legacy system remains operational, there’s no service interruption—you remain in the driving seat at all times.
We’d love to give you a demo.
Please leave us your details and we'll be in touch shortly